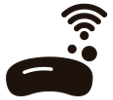 |
SoapySDR
0.8.0-gab626068
Vendor and platform neutral SDR interface library
|
Go to the documentation of this file.
309 const size_t *channels,
310 const size_t numChans,
354 const long long timeNs,
355 const size_t numElems);
375 const long long timeNs);
400 const size_t numElems,
403 const long timeoutUs);
427 const void *
const *buffs,
428 const size_t numElems,
430 const long long timeNs,
431 const long timeoutUs);
459 const long timeoutUs);
520 const long timeoutUs);
532 const size_t handle);
557 const long timeoutUs);
578 const size_t numElems,
580 const long long timeNs);
SOAPY_SDR_API SoapySDRRange * SoapySDRDevice_getFrequencyRangeComponent(const SoapySDRDevice *device, const int direction, const size_t channel, const char *name, size_t *length)
SOAPY_SDR_API char * SoapySDRDevice_getClockSource(const SoapySDRDevice *device)
#define SOAPY_SDR_API
Definition: Config.h:41
SOAPY_SDR_API double SoapySDRDevice_getReferenceClockRate(const SoapySDRDevice *device)
SOAPY_SDR_API int SoapySDRDevice_lastStatus(void)
SOAPY_SDR_API int SoapySDRDevice_writeChannelSetting(SoapySDRDevice *device, const int direction, const size_t channel, const char *key, const char *value)
SOAPY_SDR_API int SoapySDRDevice_setGainMode(SoapySDRDevice *device, const int direction, const size_t channel, const bool automatic)
SOAPY_SDR_API bool SoapySDRDevice_hasIQBalance(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API SoapySDRDevice * SoapySDRDevice_make(const SoapySDRKwargs *args)
SOAPY_SDR_API unsigned * SoapySDRDevice_readRegisters(const SoapySDRDevice *device, const char *name, const unsigned addr, size_t *length)
SOAPY_SDR_API void SoapySDRDevice_releaseReadBuffer(SoapySDRDevice *device, SoapySDRStream *stream, const size_t handle)
SOAPY_SDR_API int SoapySDRDevice_writeGPIODirMasked(SoapySDRDevice *device, const char *bank, const unsigned dir, const unsigned mask)
SOAPY_SDR_API SoapySDRRange * SoapySDRDevice_getReferenceClockRates(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API double * SoapySDRDevice_listBandwidths(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API double SoapySDRDevice_getGainElement(const SoapySDRDevice *device, const int direction, const size_t channel, const char *name)
struct SoapySDRStream SoapySDRStream
Forward declaration of stream handle.
Definition: Device.h:32
SOAPY_SDR_API unsigned SoapySDRDevice_readGPIO(const SoapySDRDevice *device, const char *bank)
SOAPY_SDR_API char ** SoapySDRDevice_listUARTs(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API char ** SoapySDRDevice_listSensors(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API double SoapySDRDevice_getFrequency(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API int SoapySDRDevice_setHardwareTime(SoapySDRDevice *device, const long long timeNs, const char *what)
SOAPY_SDR_API size_t SoapySDRDevice_getNumChannels(const SoapySDRDevice *device, const int direction)
SOAPY_SDR_API char * SoapySDRDevice_getHardwareKey(const SoapySDRDevice *device)
SOAPY_SDR_API SoapySDRStream * SoapySDRDevice_setupStream(SoapySDRDevice *device, const int direction, const char *format, const size_t *channels, const size_t numChans, const SoapySDRKwargs *args)
SOAPY_SDR_API SoapySDRDevice ** SoapySDRDevice_make_list(const SoapySDRKwargs *argsList, const size_t length)
SOAPY_SDR_API int SoapySDRDevice_readStream(SoapySDRDevice *device, SoapySDRStream *stream, void *const *buffs, const size_t numElems, int *flags, long long *timeNs, const long timeoutUs)
SOAPY_SDR_API int SoapySDRDevice_writeGPIODir(SoapySDRDevice *device, const char *bank, const unsigned dir)
SOAPY_SDR_API SoapySDRRange * SoapySDRDevice_getSampleRateRange(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API bool SoapySDRDevice_hasDCOffsetMode(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API SoapySDRDevice * SoapySDRDevice_makeStrArgs(const char *args)
SOAPY_SDR_API SoapySDRKwargs SoapySDRDevice_getHardwareInfo(const SoapySDRDevice *device)
struct SoapySDRDevice SoapySDRDevice
Forward declaration of device handle.
Definition: Device.h:29
SOAPY_SDR_API int SoapySDRDevice_deactivateStream(SoapySDRDevice *device, SoapySDRStream *stream, const int flags, const long long timeNs)
SOAPY_SDR_API bool SoapySDRDevice_hasHardwareTime(const SoapySDRDevice *device, const char *what)
SOAPY_SDR_API int SoapySDRDevice_writeUART(SoapySDRDevice *device, const char *which, const char *data)
SOAPY_SDR_API int SoapySDRDevice_getDCOffset(const SoapySDRDevice *device, const int direction, const size_t channel, double *offsetI, double *offsetQ)
SOAPY_SDR_API int SoapySDRDevice_setFrequencyComponent(SoapySDRDevice *device, const int direction, const size_t channel, const char *name, const double frequency, const SoapySDRKwargs *args)
SOAPY_SDR_API int SoapySDRDevice_acquireWriteBuffer(SoapySDRDevice *device, SoapySDRStream *stream, size_t *handle, void **buffs, const long timeoutUs)
SOAPY_SDR_API int SoapySDRDevice_setFrequencyCorrection(SoapySDRDevice *device, const int direction, const size_t channel, const double value)
Definition for a key/value string map.
Definition: Types.h:34
SOAPY_SDR_API SoapySDRKwargs * SoapySDRDevice_enumerate(const SoapySDRKwargs *args, size_t *length)
SOAPY_SDR_API char ** SoapySDRDevice_getStreamFormats(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_setGain(SoapySDRDevice *device, const int direction, const size_t channel, const double value)
SOAPY_SDR_API double SoapySDRDevice_getFrequencyComponent(const SoapySDRDevice *device, const int direction, const size_t channel, const char *name)
SOAPY_SDR_API bool SoapySDRDevice_hasIQBalanceMode(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API int SoapySDRDevice_writeGPIO(SoapySDRDevice *device, const char *bank, const unsigned value)
SOAPY_SDR_API int SoapySDRDevice_acquireReadBuffer(SoapySDRDevice *device, SoapySDRStream *stream, size_t *handle, const void **buffs, int *flags, long long *timeNs, const long timeoutUs)
SOAPY_SDR_API bool SoapySDRDevice_getGainMode(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API char * SoapySDRDevice_getAntenna(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API bool SoapySDRDevice_hasDCOffset(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API SoapySDRRange * SoapySDRDevice_getMasterClockRates(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API char * SoapySDRDevice_readSensor(const SoapySDRDevice *device, const char *key)
SOAPY_SDR_API SoapySDRRange SoapySDRDevice_getGainRange(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API char ** SoapySDRDevice_listTimeSources(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_setCommandTime(SoapySDRDevice *device, const long long timeNs, const char *what)
SOAPY_SDR_API SoapySDRRange * SoapySDRDevice_getBandwidthRange(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_setClockSource(SoapySDRDevice *device, const char *source)
SOAPY_SDR_API char ** SoapySDRDevice_listRegisterInterfaces(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_setAntenna(SoapySDRDevice *device, const int direction, const size_t channel, const char *name)
SOAPY_SDR_API bool SoapySDRDevice_hasFrequencyCorrection(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API int SoapySDRDevice_setSampleRate(SoapySDRDevice *device, const int direction, const size_t channel, const double rate)
SOAPY_SDR_API long long SoapySDRDevice_getHardwareTime(const SoapySDRDevice *device, const char *what)
SOAPY_SDR_API size_t SoapySDRDevice_getNumDirectAccessBuffers(SoapySDRDevice *device, SoapySDRStream *stream)
SOAPY_SDR_API char ** SoapySDRDevice_listChannelSensors(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API bool SoapySDRDevice_getIQBalanceMode(const SoapySDRDevice *device, const int direction, const size_t channel)
const SOAPY_SDR_API char * SoapySDRDevice_lastError(void)
SOAPY_SDR_API double SoapySDRDevice_getMasterClockRate(const SoapySDRDevice *device)
SOAPY_SDR_API int SoapySDRDevice_setFrontendMapping(SoapySDRDevice *device, const int direction, const char *mapping)
SOAPY_SDR_API char * SoapySDRDevice_getNativeStreamFormat(const SoapySDRDevice *device, const int direction, const size_t channel, double *fullScale)
Definition for a min/max numeric range.
Definition: Types.h:26
SOAPY_SDR_API char * SoapySDRDevice_readChannelSetting(const SoapySDRDevice *device, const int direction, const size_t channel, const char *key)
SOAPY_SDR_API unsigned SoapySDRDevice_transactSPI(SoapySDRDevice *device, const int addr, const unsigned data, const size_t numBits)
SOAPY_SDR_API SoapySDRKwargs * SoapySDRDevice_enumerateStrArgs(const char *args, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_getDirectAccessBufferAddrs(SoapySDRDevice *device, SoapySDRStream *stream, const size_t handle, void **buffs)
SOAPY_SDR_API char * SoapySDRDevice_readSetting(const SoapySDRDevice *device, const char *key)
SOAPY_SDR_API int SoapySDRDevice_activateStream(SoapySDRDevice *device, SoapySDRStream *stream, const int flags, const long long timeNs, const size_t numElems)
SOAPY_SDR_API SoapySDRDevice ** SoapySDRDevice_make_listStrArgs(const char *const *argsList, const size_t length)
SOAPY_SDR_API int SoapySDRDevice_writeSetting(SoapySDRDevice *device, const char *key, const char *value)
SOAPY_SDR_API unsigned SoapySDRDevice_readRegister(const SoapySDRDevice *device, const char *name, const unsigned addr)
SOAPY_SDR_API bool SoapySDRDevice_getFullDuplex(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API int SoapySDRDevice_setMasterClockRate(SoapySDRDevice *device, const double rate)
SOAPY_SDR_API int SoapySDRDevice_closeStream(SoapySDRDevice *device, SoapySDRStream *stream)
SOAPY_SDR_API unsigned SoapySDRDevice_readGPIODir(const SoapySDRDevice *device, const char *bank)
SOAPY_SDR_API int SoapySDRDevice_setReferenceClockRate(SoapySDRDevice *device, const double rate)
SOAPY_SDR_API int SoapySDRDevice_setGainElement(SoapySDRDevice *device, const int direction, const size_t channel, const char *name, const double value)
SOAPY_SDR_API int SoapySDRDevice_unmake_list(SoapySDRDevice **devices, const size_t length)
SOAPY_SDR_API double * SoapySDRDevice_listSampleRates(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_setIQBalanceMode(SoapySDRDevice *device, const int direction, const size_t channel, const bool automatic)
SOAPY_SDR_API char ** SoapySDRDevice_listClockSources(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API size_t SoapySDRDevice_getStreamMTU(const SoapySDRDevice *device, SoapySDRStream *stream)
SOAPY_SDR_API int SoapySDRDevice_setTimeSource(SoapySDRDevice *device, const char *source)
SOAPY_SDR_API SoapySDRArgInfo * SoapySDRDevice_getFrequencyArgsInfo(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API char * SoapySDRDevice_readI2C(SoapySDRDevice *device, const int addr, size_t *numBytes)
SOAPY_SDR_API void * SoapySDRDevice_getNativeDeviceHandle(const SoapySDRDevice *device)
SOAPY_SDR_API char * SoapySDRDevice_readChannelSensor(const SoapySDRDevice *device, const int direction, const size_t channel, const char *key)
SOAPY_SDR_API int SoapySDRDevice_readStreamStatus(SoapySDRDevice *device, SoapySDRStream *stream, size_t *chanMask, int *flags, long long *timeNs, const long timeoutUs)
SOAPY_SDR_API SoapySDRRange * SoapySDRDevice_getFrequencyRange(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API char ** SoapySDRDevice_listGains(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API bool SoapySDRDevice_hasGainMode(const SoapySDRDevice *device, const int direction, const size_t channel)
Definition for argument info.
Definition: Types.h:63
SOAPY_SDR_API char * SoapySDRDevice_readUART(const SoapySDRDevice *device, const char *which, const long timeoutUs)
SOAPY_SDR_API char * SoapySDRDevice_getDriverKey(const SoapySDRDevice *device)
SOAPY_SDR_API int SoapySDRDevice_setIQBalance(SoapySDRDevice *device, const int direction, const size_t channel, const double balanceI, const double balanceQ)
SOAPY_SDR_API SoapySDRArgInfo * SoapySDRDevice_getStreamArgsInfo(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_writeStream(SoapySDRDevice *device, SoapySDRStream *stream, const void *const *buffs, const size_t numElems, int *flags, const long long timeNs, const long timeoutUs)
SOAPY_SDR_API void SoapySDRDevice_releaseWriteBuffer(SoapySDRDevice *device, SoapySDRStream *stream, const size_t handle, const size_t numElems, int *flags, const long long timeNs)
SOAPY_SDR_API int SoapySDRDevice_writeRegister(SoapySDRDevice *device, const char *name, const unsigned addr, const unsigned value)
SOAPY_SDR_API SoapySDRArgInfo * SoapySDRDevice_getChannelSettingInfo(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_writeI2C(SoapySDRDevice *device, const int addr, const char *data, const size_t numBytes)
SOAPY_SDR_API int SoapySDRDevice_writeRegisters(SoapySDRDevice *device, const char *name, const unsigned addr, const unsigned *value, const size_t length)
SOAPY_SDR_API char ** SoapySDRDevice_listAntennas(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_setDCOffsetMode(SoapySDRDevice *device, const int direction, const size_t channel, const bool automatic)
SOAPY_SDR_API SoapySDRArgInfo * SoapySDRDevice_getSettingInfo(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API double SoapySDRDevice_getFrequencyCorrection(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API SoapySDRArgInfo SoapySDRDevice_getSensorInfo(const SoapySDRDevice *device, const char *key)
SOAPY_SDR_API char * SoapySDRDevice_getFrontendMapping(const SoapySDRDevice *device, const int direction)
SOAPY_SDR_API int SoapySDRDevice_writeGPIOMasked(SoapySDRDevice *device, const char *bank, const unsigned value, const unsigned mask)
SOAPY_SDR_API int SoapySDRDevice_setFrequency(SoapySDRDevice *device, const int direction, const size_t channel, const double frequency, const SoapySDRKwargs *args)
SOAPY_SDR_API SoapySDRRange SoapySDRDevice_getGainElementRange(const SoapySDRDevice *device, const int direction, const size_t channel, const char *name)
SOAPY_SDR_API int SoapySDRDevice_setBandwidth(SoapySDRDevice *device, const int direction, const size_t channel, const double bw)
SOAPY_SDR_API int SoapySDRDevice_setDCOffset(SoapySDRDevice *device, const int direction, const size_t channel, const double offsetI, const double offsetQ)
SOAPY_SDR_API SoapySDRKwargs SoapySDRDevice_getChannelInfo(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API double SoapySDRDevice_getBandwidth(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API int SoapySDRDevice_unmake(SoapySDRDevice *device)
SOAPY_SDR_API double SoapySDRDevice_getSampleRate(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API char ** SoapySDRDevice_listFrequencies(const SoapySDRDevice *device, const int direction, const size_t channel, size_t *length)
SOAPY_SDR_API char ** SoapySDRDevice_listGPIOBanks(const SoapySDRDevice *device, size_t *length)
SOAPY_SDR_API int SoapySDRDevice_getIQBalance(const SoapySDRDevice *device, const int direction, const size_t channel, double *balanceI, double *balanceQ)
SOAPY_SDR_API bool SoapySDRDevice_getDCOffsetMode(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API SoapySDRArgInfo SoapySDRDevice_getChannelSensorInfo(const SoapySDRDevice *device, const int direction, const size_t channel, const char *key)
SOAPY_SDR_API double SoapySDRDevice_getGain(const SoapySDRDevice *device, const int direction, const size_t channel)
SOAPY_SDR_API char * SoapySDRDevice_getTimeSource(const SoapySDRDevice *device)