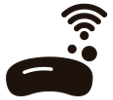 |
SoapySDR
0.8.0-gab626068
Vendor and platform neutral SDR interface library
|
Go to the documentation of this file.
14 #include <type_traits>
23 typedef std::map<std::string, std::string>
Kwargs;
47 template <
typename Type>
57 template <
typename Type>
71 Range(
const double minimum,
const double maximum,
const double step=0.0);
74 double minimum(
void)
const;
77 double maximum(
void)
const;
80 double step(
void)
const;
83 double _min, _max, _step;
123 enum Type {BOOL, INT, FLOAT, STRING} type;
173 template <
typename Type>
174 typename std::enable_if<std::is_same<Type, bool>::value, Type>::type
StringToSetting(
const std::string &s)
180 if (s ==
"0")
return false;
181 if (s ==
"0.0")
return false;
182 if (s ==
"")
return false;
188 template <
typename Type>
189 typename std::enable_if<not std::is_same<Type, bool>::value and std::is_integral<Type>::value and std::is_signed<Type>::value, Type>::type
StringToSetting(
const std::string &s)
191 return Type(std::stoll(s));
194 template <
typename Type>
195 typename std::enable_if<not std::is_same<Type, bool>::value and std::is_integral<Type>::value and std::is_unsigned<Type>::value, Type>::type
StringToSetting(
const std::string &s)
197 return Type(std::stoull(s));
200 template <
typename Type>
201 typename std::enable_if<std::is_floating_point<Type>::value, Type>::type
StringToSetting(
const std::string &s)
203 return Type(std::stod(s));
206 template <
typename Type>
207 typename std::enable_if<std::is_same<typename std::decay<Type>::type, std::string>::value, Type>::type
StringToSetting(
const std::string &s)
227 template <
typename Type>
230 return std::to_string(s);
235 template <
typename Type>
238 return SoapySDR::Detail::StringToSetting<Type>(s);
241 template <
typename Type>
#define SOAPY_SDR_API
Definition: Config.h:41
Type
The data type of the argument (required)
Definition: Types.hpp:123
#define SOAPY_SDR_FALSE
String definition for boolean false used in settings.
Definition: Types.h:23
std::string SettingToString(const Type &s)
Definition: Types.hpp:242
#define SOAPY_SDR_TRUE
String definition for boolean true used in settings.
Definition: Types.h:20
std::enable_if< std::is_same< Type, bool >::value, Type >::type StringToSetting(const std::string &s)
Definition: Types.hpp:174
double step(void) const
Get the range step size.
Definition: Types.hpp:162
double maximum(void) const
Get the range maximum.
Definition: Types.hpp:157
double minimum(void) const
Get the range minimum.
Definition: Types.hpp:152
std::vector< Range > RangeList
Definition: Types.hpp:91
std::string key
The key used to identify the argument (required)
Definition: Types.hpp:104
Definition: ConverterPrimitives.hpp:14
std::vector< ArgInfo > ArgInfoList
Definition: Types.hpp:148
std::string SettingToString(const bool &s)
Definition: Types.hpp:212
SOAPY_SDR_API Kwargs KwargsFromString(const std::string &markup)
Type StringToSetting(const std::string &s)
Definition: Types.hpp:236
std::map< std::string, std::string > Kwargs
Typedef for a dictionary of key-value string arguments.
Definition: Types.hpp:23
std::vector< std::string > options
Definition: Types.hpp:136
std::string description
A brief description about the argument (optional)
Definition: Types.hpp:117
std::vector< Kwargs > KwargsList
Typedef for a list of key-word dictionaries.
Definition: Types.hpp:38
SOAPY_SDR_API std::string KwargsToString(const Kwargs &args)
std::vector< std::string > optionNames
Definition: Types.hpp:142
std::string value
Definition: Types.hpp:111
Range range
Definition: Types.hpp:130
std::string units
The units of the argument: dB, Hz, etc (optional)
Definition: Types.hpp:120
std::string name
The displayable name of the argument (optional, use key if empty)
Definition: Types.hpp:114